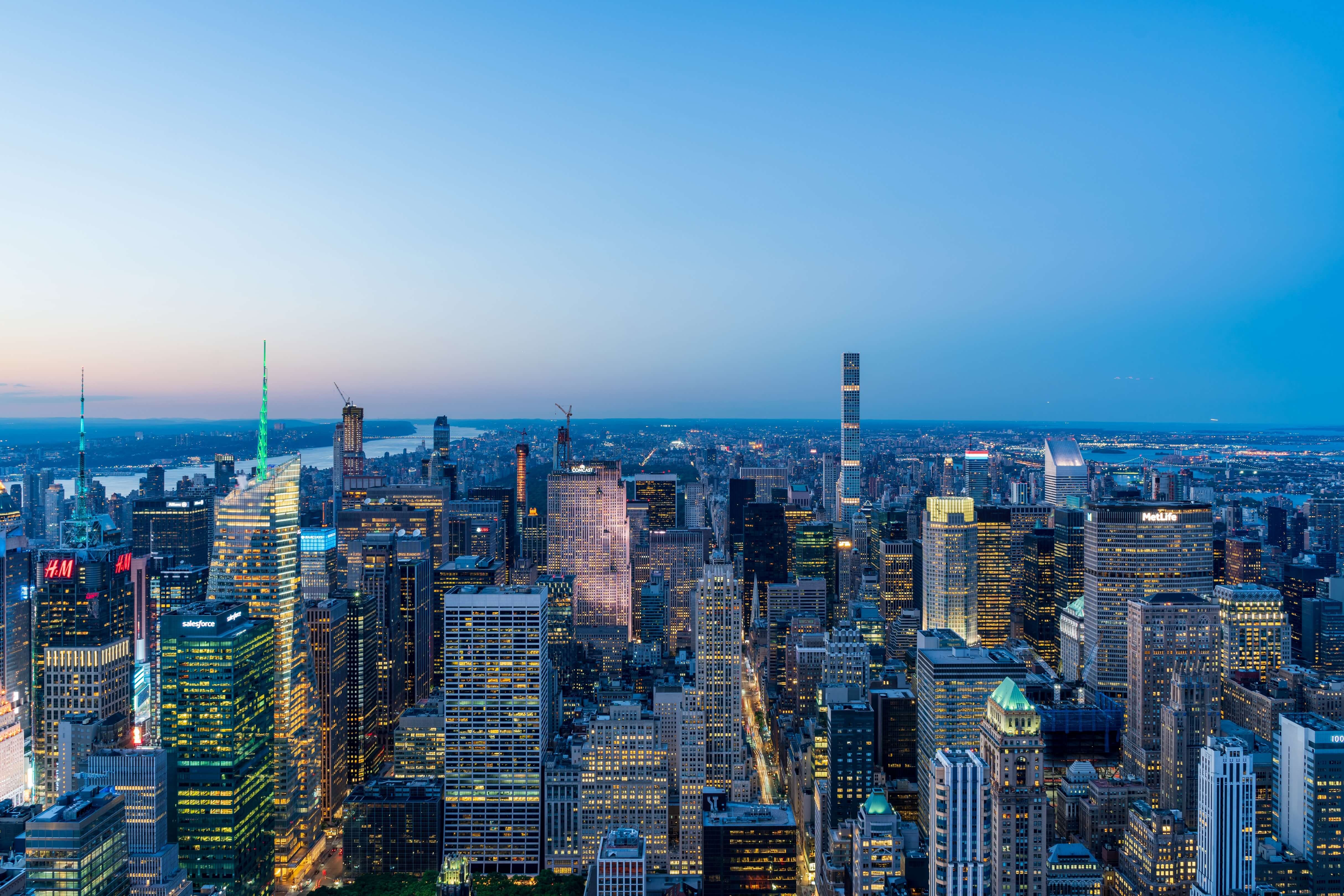
React Native: Use Lottie as Splash Screen
Here's a step-by-step to setup Lottie as splash screen in React Native app
1. Create New React Native Project
Let's start by create a new project & install lottie-react-native & lottie-ios@3.2.3
don't forget to do this one if youre gonna run the code as ios app
2. Cleanup Existing App.js
For clarity, let's cleanup App.js, then replace the content with this one
import React from 'react'; import {Text, View, StyleSheet} from 'react-native'; const App = () => { return ( <View style={styles.text}> <Text> Hello Lottie Splash! </Text> </View> ); }; const styles = StyleSheet.create({ text: { alignItems: 'center', flex: 1, justifyContent: 'center', fontWeight: 'bold', }, }); export default App;
3. Download & Save Lottie Files
You can pick whatever lottie you like, see https://lottiefiles.com/featured for free Lottie files. I'm gonna use this one for the splash screen :
https://lottiefiles.com/61851-eid-al-fitr-eid-mubarak-2021
Download the lottie as json file, then save it in (you can add assets
folder inside the project)
assets/eid-mubarrak-lottie.json
4. Display Lottie Component in View
Let's display Lottie components in our view
import React from 'react'; import {Text, View, StyleSheet} from 'react-native'; import LottieView from 'lottie-react-native'; const App = () => { return ( <View style={styles.text}> <LottieView source={require('./assets/eid-mubarak-lottie.json')} autoPlay loop={false} /> <Text> Hello Lottie Splash! </Text> </View> ); }; const styles = StyleSheet.create({ text: { alignItems: 'center', flex: 1, justifyContent: 'center', fontWeight: 'bold', }, }); export default App;
Run the code, here's mine when running using ios simulator
npx react-native run-ios --simulator="iPhone SE (3rd generation)"
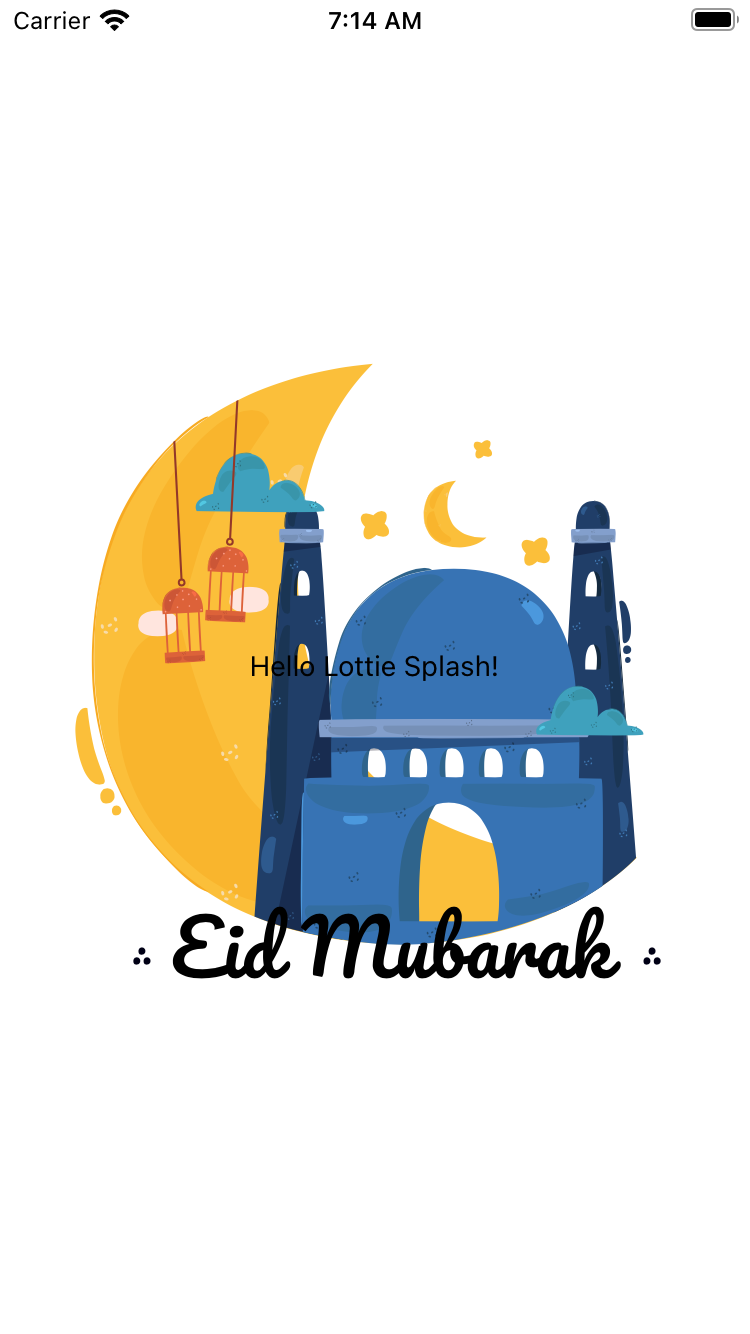
You can see that it will display animation whenever we open the application, but we need to hide that after animation complete.
5. Configure as Splash Screen
onAnimationFinish
props, the main idea : we display the lottie animation first, after animation completed, we can display our main content.We can use react-hook state to accomplish this one
import React, {useState} from 'react'; import {Text, View, StyleSheet} from 'react-native'; import LottieView from 'lottie-react-native'; const App = () => { const [lottieLoaded, setLottieLoaded] = useState(false); if (!lottieLoaded) { return ( <View style={styles.text}> <LottieView source={require('./assets/eid-mubarak-lottie.json')} autoPlay loop={false} resizeMode="contain" onAnimationFinish={() => { setLottieLoaded(true); }} /> </View> ); } return ( <View style={styles.text}> <Text> Hello Lottie Splash! </Text> </View> ); }; const styles = StyleSheet.create({ text: { alignItems: 'center', flex: 1, justifyContent: 'center', fontWeight: 'bold', }, }); export default App;
Here's the result, it will display the lottie animation, once animation completed, it will display the main content.
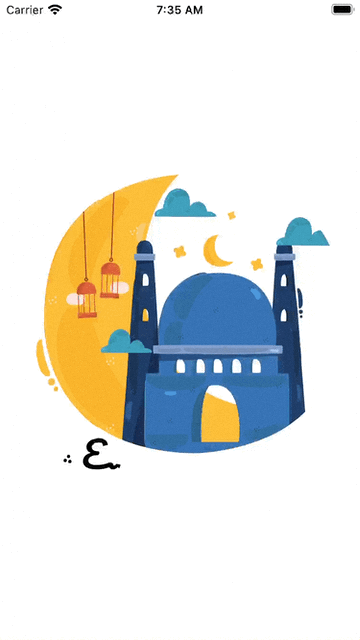
That's all.
Happy Eid Mubarak Everyone!
Comments