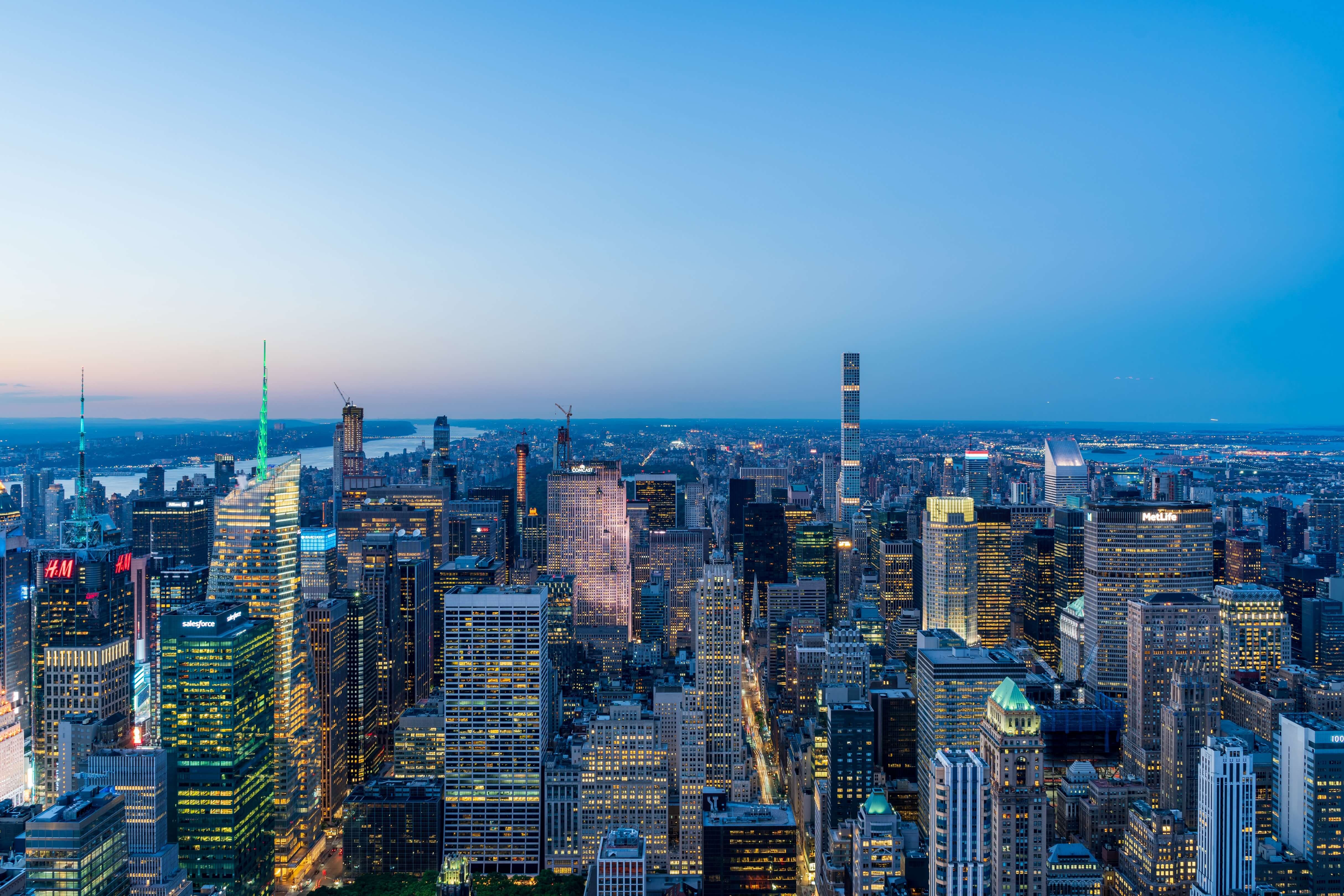
Flutter Tips : Formatting Code
Widget based code on flutter often resulting a deep tree-shaped structure. That kind of structure. Slowly but sure. Will definitely kill you when your codebase becoming larger and larger.
Fortunately flutter already give us an awesome formatter. However, the awesomeness of flutter formatter will only working under 1 condition : when we use optional trailing commas.
Always add a trailing comma at the end of a parameter list in functions, methods, and constructors.
For example, flutter format code will have no effect on the following codes
import 'package:flutter/material.dart';
void main() => runApp(
MaterialApp(home: HiPage()),
);
class HiPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Hi();
}
}
class Hi extends StatefulWidget {
@override
_HiState createState() => _HiState();
}
class _HiState extends State {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar:
AppBar(title: Text('Hi Flutter'), backgroundColor: Colors.blue[900]),
body: Container(
color: Colors.blue,
child: Center(
child: Text('Hi Flutter',
style: TextStyle(color: Colors.white, fontSize: 50)),
),
),
);
}
}
After some checking. You could notice that, we are missing optional trailing commas on the following lines1. AppBar(title: Text('Hi Flutter'), backgroundColor: Colors.blue[900]),
2. style: TextStyle(color: Colors.white, fontSize: 50)),
with only adding optional training commas on the following lines1. AppBar(title: Text('Hi Flutter'), backgroundColor: Colors.blue[900], ),
2. style: TextStyle(color: Colors.white, fontSize: 50,),),
And the run the formatter, our code will be like this.
import 'package:flutter/material.dart';
void main() => runApp(
MaterialApp(home: HiPage()),
);
class HiPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Hi();
}
}
class Hi extends StatefulWidget {
@override
_HiState createState() => _HiState();
}
class _HiState extends State {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Hi Flutter'),
backgroundColor: Colors.blue[900],
),
body: Container(
color: Colors.blue,
child: Center(
child: Text(
'Hi Flutter',
style: TextStyle(
color: Colors.white,
fontSize: 50,
),
),
),
),
);
}
}
Neat right? Just keep that in mind :
Always add a trailing comma at the end of a parameter list in functions, methods, and constructors.
That's all
Comments